SMALL
1. Express 웹 프레임워크
웹 서버를 생성하고 HTTP 요청에 대한 라우팅 및 처리, 미들웨어를 통한 요청 및 응답 처리 등을 간단하게 구현할 수 있음
다양한 확장 기능과 모듈을 제공하여 개발 생산성을 높일 수 있음
- express 모듈 설치
npm i express
<<<<json 수정하기>>>> - [package.json] "type":"module" "start":"nodemon 7_express <<<<npm 실행방법>>>> - [terminal] npm start |
- 미들웨어
< js >
import express from 'express';
//객체 생성
const app = express();
//미들웨어
//next: 다음 미들웨어를 호출
app.use((req,res,next)=>{
res.setHeader('node-skill','node.js come on!');
next();
});
app.get('/',(req,res,next) =>{
res.send('<h2> 익스프레스 서버로 만든 첫번째 페이지</h2>');
next();
});
app.get('/hello', (req, res, next)=> {
res.setHeader('Content-Type','application/json');
res.status(200).json({userid:'apple',name:'김사과',age:20});
});
//서버 발생
app.listen(8080);
</js >
![]() ![]() |
2. get
- get_1
< js >
import express from 'express';
const app=express();
// localhost:8080/posts
// localhost:8080/posts?number=1
// localhost:8080/posts?number=1&userid=apple
app.get('/posts',(req,res)=>{
console.log('posts 호출');
console.log('path: ' , req.path);
console.log('query: ' , req.query);
res.sendStatus(200);
});
app.listen(8080);
</js >
![]() [terminal] posts 호출 path: /posts query: {} ------------------------------------------------------------------------------ ![]() [terminal] posts 호출 path: /posts query: { number: '1' } |
- get_2
< js >
import express from 'express';
const app=express();
// localhost:8080/posts/1 -> params: { id: '1' }
// localhost:8080/posts/1?id=10
// get('/posts/:id')에서 id는 임의로 정한 변수이다. (꼭 id라고 적지 않아도 됨)
app.get('/posts/:id',(req, res)=>{
console.log('/posts/:id 호출!!');
console.log('path: ',req.path);
console.log('query: ',req.query);
console.log('params: ',req.params);
res.sendStatus(200);
});
app.listen(8080);
</js >
![]() [terminal] ![]() |
- get_3
< js >
import express from 'express';
const app=express();
// localhost:8080/mypage
// localhost:8080/myroom
app.get(['/mypage','/myroom'],(req,res) =>{
res.send('<h2>mypage&myroom</h2>');
});
app.listen(8080);
</js >
![]() ![]() |
- get_4
< js >
import express from 'express';
const app=express();
// localhost:8080/10
app.get('/:userid',(req,res)=>{
console.log(req.path, '호출!');
console.log(`${req.params.userid}번 멤버가 출동!`);
res.sendStatus(200);
});
app.listen(8080);
</js >
![]() [terminal] /10 호출! 10번 멤버가 출동! |
- get_5
< js >
import express from 'express';
const app=express();
// localhost:8080/member/10
app.get('/member/:userid',(req, res) => {
console.log(req.path, '호출');
console.log(`${req.params.userid}번 멤버가 출력됨!`);
res.sendStatus(200);
});
app.listen(8080);
</js >
![]() |
- ejs 활용하기
< login.ejs >
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>로그인</title>
</head>
<body>
<h2>로그인</h2>
<form action="/login" method="get"></form>
<p>아이디: <input type="text" name="userid" id="userid"></p>
<p>비밀번호: <input type="password" name="userpw" id="userpw"></p>
<p><button type="submit">로그인</button></p>
</body>
</html>
</login.ejs >
< js >
import express from 'express';
const app=express();
// http://localhost:8080/
// http://localhost:8080/login?userid=apple&userpw=1234
app.get('/', (req, res) => {
res.send(`
<h2>로그인</h2>
<form action="/login" method="get">
<p>아이디: <input type="text" name="userid" id="userid"></p>
<p>비밀번호: <input type="password" name="userpw" id="userpw"></p>
<p><button type="submit">로그인</button></p>
</form>
`);
});
// localhost:8080/posts
// localhost:8080/posts?id=1
// localhost:8080/posts?id=1&userid=apple&name=김사과
app.get('/posts',(req,res) => {
console.log('posts를 호출');
console.log('path: ', req.path);
console.log('query: ', req.query);
res.sendStatus(200);
});
// localhost:8080/mypage
// localhost:8080/myroom
app.get(['/mypage','/myroom'],(req,res) =>{
res.send('<h2>mypage&myroom</h2>');
});
// localhost:8080/member/10
// { userid: 10 }
app.get('/member/:userid',(req, res) => {
console.log(req.path, '호출');
console.log(`${req.params.userid}번 멤버가 출력됨!`);
res.sendStatus(200);
});
// http://localhost:8080/login?userid=apple&userpw=1111
app.get('/login',(req,res) => {
console.log('login 호출');
console.log('path: ', req.path);
console.log('query: ', req.query);
res.sendStatus(200);
});
app.listen(8080);
</js >
![]() ![]() |
- post
설치: npm i body parser
< js >
import express from 'express';
import bodyParser from 'body-parser';
const app = express();
app.use(bodyParser.urlencoded({ extended: true }));
// http://localhost:8080/
// http://localhost:8080/login?userid=apple&userpw=1234
app.get('/', (req, res) => {
res.send(`
<h2>로그인</h2>
<form action="/login" method="post">
<p>아이디: <input type="text" name="userid" id="userid"></p>
<p>비밀번호: <input type="password" name="userpw" id="userpw"></p>
<p><button type="submit">로그인</button></p>
</form>
`);
});
// http://localhost:8080/login?userid=apple&userpw=1111
app.post('/login', (req, res) => {
console.log('POST /login 호출');
console.log('body: ', req.body);
res.status(200).send('로그인 되었습니다!');
});
app.listen(8080);
</js >
[terminal] POST /login 호출 body: { userid: 'apple', userpw: '1111' } ![]() |
- post man: https://www.postman.com/downloads/
Download Postman | Get Started for Free
Try Postman for free! Join 30 million developers who rely on Postman, the collaboration platform for API development. Create better APIs—faster.
www.postman.com
더보기
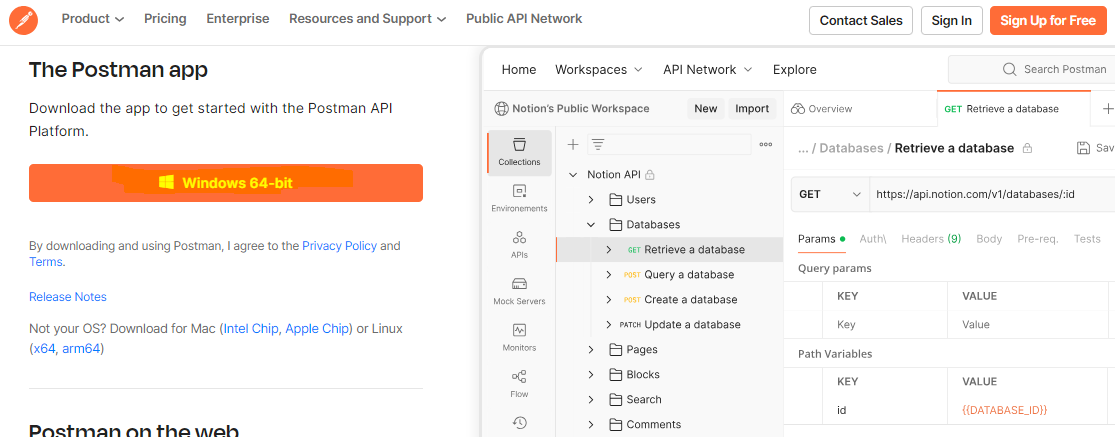
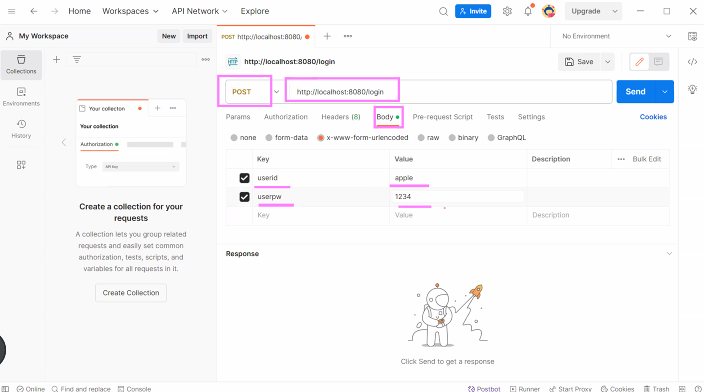
- 설치하고 가입
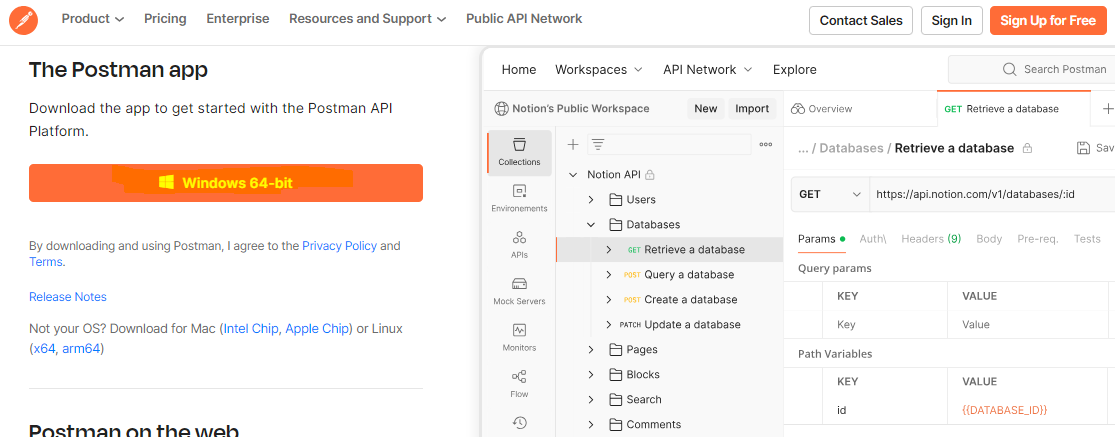
- post 주소 입력
Key:Value 입력
전송
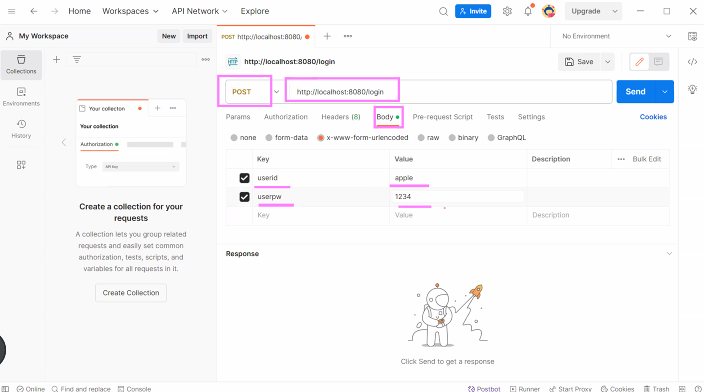
3. post
- Package.json 활용
1. 새로운 작업 파일에 package-json 복사
2. [terminal] npm i 입력
3. package-json 세가지 변경
- post 글 올리기
< js >
import express from 'express';
const app=express();
// 미들웨어에 등록
app.use(express.json());
app.post('/posts',(req,res)=>{
console.log(req.body);
res.status(201).send('글이 새로 등록되었어요');
});
app.listen(8080);
</js >
![]() ![]() |
4. error
- error_1 : 비동기식
// 에러 핸들링
import express from 'express';
import fs from 'fs';
const app=express();
app.use(express.json());
// localhost:8080/file1
// 비동기식
app.get('/file1',(req,res)=>{
fs.readFile('/file1.txt',(err,data)=>{ // 파일이 없어서 백퍼 에러가 난다
if(err){
res.sendStatus(404);
}
});
});
app.listen(8080);
![]() |
- error_2 : 동기식
< js >
// 에러 핸들링
import express from 'express';
import fs from 'fs';
const app=express();
app.use(express.json());
// localhost:8080/file2
// file2를 읽어 에러가 발생하면 404를 리턴
// 단 file2를 읽을 경우 동기식으로 작성
app.get('/file2',(req,res)=>{
try{
const data=fs.readFileSync('/file2.txt');
}catch(error){
res.sendStatus(404);
}
})
app.listen(8080);
</js >
![]() |
- error_3
: 비동기식 | 비동기식 -> 동기식처럼 진행
< js >
//파일 시스템을 비동기로 처리하는 모듈
import fsAsync from 'fs/promises'
// 비동기
app.get('/file3',(req,res)=>{
fsAsync.readFile('/file3.txt')
.catch((error)=>{
res.sendStatus(404);
})
});
// 비동기로 하는 일들을 동기처럼 차례대로 실행
// async ~ await: 비동기 프로그래밍에서 async 함수 내에서 Promise를 처리할 때 사용.
await를 사용하면 프로그램은 Promise가 완료될때까지 기다리며
그 후 비동기 작업의 결과를 반환하거나 처리
// Promise: 얘는 좀 처리하고 가겠다고 약속(?)하는 기능...?
app.get('/file4',async (req,res)=>{
try{
const data=await fsAsync.readFile('/file4.txt');
}catch(error){
res.sendStatus(404);
}
});
app.listen(8080);
</js >
![]() ![]() |
- error_4
< js >
import express from 'express';
const app=express();
app.use((req,res,next)=>{
const error=new Error('테스트 에러!!');
next(error);
})
app.use((error,req,res,next)=>{
console.error(error);
res.status(500).json({message:'서버 에러!!'});
})
app.listen(8080);
</js >
/file5 ![]() ![]() |
5. route( )
특정 URL에 대한 미들 웨어 및 라우팅 로직을 모듈화하고 그룹화 할 수 있음
가독성을 향상 시키고 유지 보수를 용이하게 함
route( ) 메서드는 Router 객체를 반환, 해당 객체로 특정 URL 경로에 대한 라우팅을 설정
- route_1
< js >
import express from 'express';
const app = express();
app
.route('/posts')
.get((req,res)=>{
res.status(200).send('GET: /posts');
})
.post((req,res)=>{
res.status(200).send('POST: /posts');
});
app.listen(8080);
</js >
![]() ![]() |
- route_2
< js >
import express from 'express';
const app = express();
app
.route('/member/:id')
.put((req, res) => {
res.status(201).send('PUT: /member/:id');
})
.delete((req, res) => {
res.status(200).send('DELETE: /member/:id');
});
app.listen(8080);
</js >
![]() ![]() |
6. morgan
http 요청에 대한 로깅을 수행하기 위한 미들웨어
express와 함께 사용되며 클라이언트로부터 오는 각각의 요청에 대한 정보를 로깅
요청 HTTP 메서드, 발생한 URL, IP 주소, 응답상태코드, 데이터의 크기를 알 수 있음
옵션: common, short, tiny, dev, combined
- 설치
mpn i morgan
- routes > uesr.js 생성
- routing
< 4_routing.js >
import express from 'express';
import morgan from 'morgan';
import userRouter from './routes/user.js';
const app=express();
app.use(express.json());
app.use(morgan('combined'));
// http://localhost:8080/users
app.use('/users', userRouter);
app.listen(8080);
</ 4_routing.js >
<user.js >
import express from 'express';
const router = express.Router();
router.use((req,res, next)=>{
console.log('users에 존재하는 미들웨어!')
next();
})
//http://locathost:8080/users(GET)
//회원정보를 보기
router.get('/',(rep, res)=>{
res.status(200).send('GET: /users 회원정보보기');
});
//http://locathost:8080/users(POST)
//회원가입
router.post('/',(rep, res)=>{
res.status(201).send('POST: /users 회원가입');
});
//http://locathost:8080/users(PUT)
//정보수정
router.put('/:id',(rep, res)=>{
res.status(201).send('PUT: /users/:id 정보수정');
});
//http://locathost:8080/users(DELETE))
//회원탈퇴
router.delete('/:id',(rep, res)=>{
res.status(201).send('DELETE: /users/:id 회원탈퇴');
});
export default router; //중요! 외부에서 사용할 수 있게
<user.js >
![]() ![]() ![]() ![]() |
- 문제
: posts 라우터 생성 후 post.js에서 아래와 같은 메서드를 테스트하자
: 글보기(GET) 글작성(POST) 글수정(PUT) 글삭제(DELETE)
- routes > post.js 생성
- 4_routing.js
< 4_routing.js >
import express from 'express';
import morgan from 'morgan'; // npm i morgan
import userRouter from './routes/user.js';
import postRouter from './routes/post.js';
const app=express();
app.use(express.json());
app.use(morgan('combined'));
// http://localhost:8080/users
app.use('/users', userRouter);
// http://localhost:8080/posts
app.use('/posts', postRouter);
app.listen(8080);
</ 4_routing.js >
- router > post.js
< post.js >
import express from 'express';
const router=express.Router();
router.use((req,res,next)=>{
console.log('posts에 존재하는 미들웨어!');
next();
});
// 글보기
router.get('/',(req,res)=>{
res.status(200).send('GET: /posts');
});
// 글작성
router.post('/',(req,res)=>{
res.status(201).send('POST: /posts');
});
// 글수정
router.put('/:id',(req,res)=>{
res.status(201).send('PUT: /posts/:id');
});
// 글삭제
router.delete('/:id',(req,res)=>{
res.status(201).send('DELETE: /posts/:id');
});
export default router;
</post.js >
![]() ![]() ![]() ![]() |
'Web > Node.js' 카테고리의 다른 글
10. 실습: Tweet (0) | 2024.04.26 |
---|---|
09. git 설치, github 간단 연동 (0) | 2024.04.25 |
07. http 모듈, 템플릿 엔진 (0) | 2024.04.24 |
06. 버퍼(buffer), 스트림(Steam) (0) | 2024.04.24 |
05. Console, This, file (0) | 2024.04.23 |